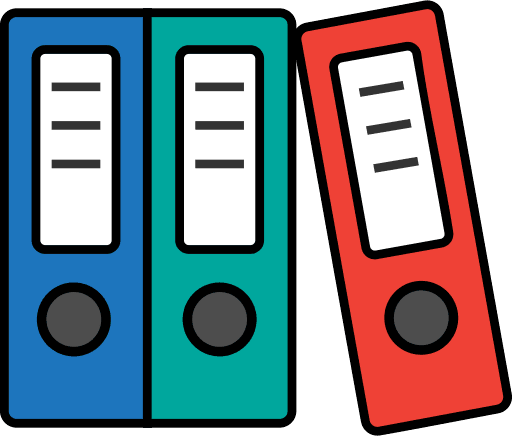
Check out the Design Patterns Explained Simply e-book!
Factory pattern explained simply
Definition
The factory pattern is a creational design pattern that helps when you have to create many different types of many different objects.
Real-World Example
Let’s think of the word “factory” in a real-world sense. A (real) factory is a building where things are manufactured. Well, in the programming sense, a factory is just an object that creates (or manufactures) other objects.
The factory design pattern allows us to handle all of our object creation in a centralized location (in the factory). Objects are created in a factory via factory methods - rather than by calling a constructor.
Get Started
Let’s say I run a technology company. In my technology company I have employees. I have 2 types of employees. There are developers who write code for new features and there are testers who test the features. We need to create an efficient way to create and store these employees.
Let’s start by creating our constructor functions - one for Developer and one for Tester:
function Developer(name)
{
this.name = name;
this.type = "Developer";
}
function Tester(name)
{
this.name = name;
this.type = "Tester";
}
We have a Developer and a Tester which both take in a name parameter, name
. They also have a type
field that represents the appropriate types - one type for “Developer” and one type for “Tester”.
Now that we have our employee constructor functions, let’s create our employee factory:
function EmployeeFactory()
{
this.create = (name, type) => {
switch(type)
{
case 1:
return new Developer(name);
case 2:
return new Tester(name);
}
}
}
This is our employee factory! We have a single method - create()
which is responsible for creating our different types of employees. create()
takes in a name which represents the name of the employee we are about to create. create()
also takes in a type
parameter that tells our factory which type of employee we want to create. In this case, Type 1 employees are going to be Developers while Type 2 employees are going to be Testers. We can see this in action in our switch statement. If type
is 1, we return a new Developer with the name that we passed in to create()
. If type
is 2, we return a new Tester with the name that we passed in.
So at this point we have our employee constructor functions for Developer and Tester and we have our employee factory constructor function. So now let’s see how to use this factory.
Let’s create a new instance of our EmployeeFactory
:
const employeeFactory = new EmployeeFactory();
const employees = [];
We’ve just created our employee factory. We’ve also created an employees
array to store all of the new employees we want to create. We can pretend this array is the database to store employees in.
Let’s say we wanted to create a new Developer named “Patrick” and store him in our employee “database”. Here’s how we do it:
employees.push(employeeFactory.create("Patrick", 1));
We’re pushing a new employee to our employees
array. But instead of creating the developer directly, we call the create()
method on our employeeFactory
. We pass in the information about the employee we want to create - name: “Patrick”, type: 1 (Developer).
Cool. So lets add some more employees to our employees
array. Let’s create 2 Developers - one named “Jamie” and the other named “Taylor”. Let’s also create 2 Testers - one named “John” and the other named “Tim”.
employees.push(employeeFactory.create("Patrick", 1));
employees.push(employeeFactory.create("John", 2));
employees.push(employeeFactory.create("Jamie", 1));
employees.push(employeeFactory.create("Taylor", 1));
employees.push(employeeFactory.create("Tim", 2));
We just created 4 new employees with our employee factory and saved them into our employees database. But how can we test this to see if it’s actually working? Let’s test this out by giving our employees a say()
method that will allow them to introduce themselves. The employees will say their name and what type of employee they are.
Let’s create our say()
method:
function say()
{
console.log("Hi, I am " + this.name + " and I am a " + this.type);
}
Our plan is to loop through all of the employees in the employees
array and call say()
for each of the employees. This will give every employee a chance to introduce themselves.
Let’s loop through our employees and call say()
on each of them:
employees.forEach( emp => {
say.call(emp);
});
The code above is looping through all employees and calling say()
for each employee, using each employee as the this reference for that call. This results in the following output:
"Hi, I am Patrick and I am a Developer"
"Hi, I am Jamie and I am a Developer"
"Hi, I am Taylor and I am a Developer"
"Hi, I am John and I am a Tester"
"Hi, I am Tim and I am a Tester"
Neat. So for each employee we created, we call say()
for each employee and print out it’s information.
Summary
The factory pattern is a creational design pattern that makes it easy to create many different types of many different objects via an object factory. A factory, in the programming sense, is just an object that creates (or manufactures) different objects.
Video
Here is a YouTube video explanation of the factory pattern.
Code
You can find the code for this tutorial on Github.